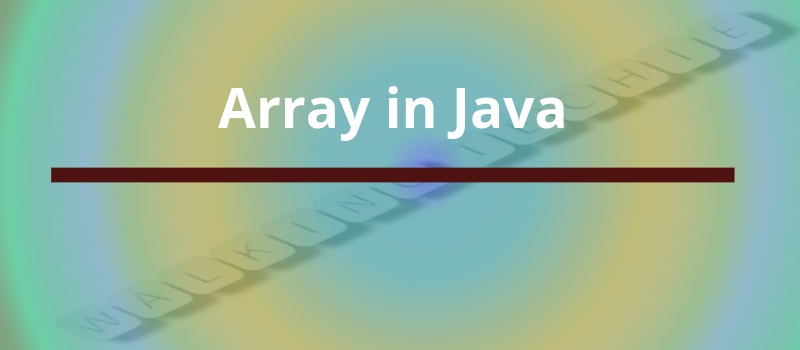
An array is a group of like-typed variables that are referred to by a common name. Arrays of any type can be created and may have one or more dimensions. A specific element in an array is accessed by its index.
One-Dimensional Arrays
The general form of a one-dimensional array declaration is
type var-name[ ]; or type[] var-name;
An array declaration has two components: the type and the name. type declares the element type of the array. The element type determines the data type of each element that comprises the array. Like array of int type, we can also create an array of other primitive data types like char, float, double etc.. or user defined data type(objects of a class).Thus, the element type for the array determines what type of data the array will hold.
// both declaration are valid int ia[]; int[] ia2;
Although this declaration establishes the fact that ia is an array variable, no
array actually exists. To link ia with an actual, physical array of integers, you must
allocate one using new
and assign it to ia. new
is a special operator that
allocates memory.
Initiating the one dimensional Array in Java
When an array is declared, only reference of an array is created. To actually create the physical form of an array, need to allocate memory to array. The general form of new as it applies to one-dimensional arrays appears as follows:
Here, type specifies the type of data being allocated, size specifies the number of elements in
array, and array-var is the variable name of array. The new
is used to allocate the memory
to an array.
The elements of an array allocated by new
will automatically initialized to zero (for numeric type),
false (for boolean), and null
(for reference types).
Example:
int intArray[] = new int[10];OR
int intArray[]; // declare an array intArray = new int[10]; // initialize an array
After the above statement executes, intArray will refer to an array of 10 elements. Further, all element in the array will be initialized to zero.
Creating an array in java is two step process. First, you must declare a variable of desired data type. Second, you must allocate the memory that will hold the array, using new, and assign to an array variable.
In Java, all arrays are dynamically allocated.
The element of the array can access by using the index inside the square brackets. All array indexes start at zero.
public class ArrayDemo { public static void main(String[] args) { int month_days[]; month_days = new int[12]; month_days[0] = 31; month_days[1] = 28; month_days[2] = 31; month_days[3] = 30; month_days[4] = 31; month_days[5] = 30; month_days[6] = 31; month_days[7] = 31; month_days[8] = 30; month_days[9] = 31; month_days[10] = 30; month_days[11] = 31; System.out.println("April has " + month_days[3] + " days."); } }
Output of the program:
April has 30 days.
Array literals
Array can be initialized when they are declared. An array initializer is a list of comma-separated expressions surrounded by curly braces. The array will automatically be created large enough to hold the number of elements you specify in the array initializer.
There is no need to use new.
// An improved version of the previous program. class ArrayDemo { public static void main(String args[]) { int month_days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31}; System.out.println("April has " + month_days[3] + " days."); } }
Java strictly checked the range of array when you try to store or reference value and access the array element.
For example, the run-time system will check the value of each index into month_days to make sure that it is between 0 and 11 inclusive.
If you try to access elements outside the range of the array (negative numbers or numbers greater than the length of the array), you will get a run-time error(ArrayIndexOutOfBoundsException).
Accessing elements of array using for loop
Lets create an example that demonstrate average of elements of array.
Refer: For loop in Java in detail.
public class Average { public static void main(String args[]) { double nums[] = {10.1, 11.2, 12.3, 13.4, 14.5}; double result = 0; int i; int length = nums.length; // for loop for (i = 0; i < length; i++) result = result + nums[i]; System.out.println("Average is " + result / length); result = 0; // foreach for (double num : nums) { result = result + num; } System.out.println("Average is " + result / length); } }
Arrays are object in Java so that we can find the length of array using the length member.
Output of the above program:
Average is 12.299999999999999 Average is 12.299999999999999
Multidimensional Arrays
In Java, multidimensional arrays are actually arrays of arrays. To declare a multidimensional array variable, specify each additional index using another set of square brackets([]).
int twoD[][]= new int[3][5]; // create 3 by 5 array(2D array) int threeD[][][]=new int[3][4][5]; // create 3 by 4 by 5 array(3D array)
public class TwoDArray { public static void main(String[] args) { int twoD[][] = new int[3][3]; int i, j, k = 0; for (i = 0; i < 3; i++) { for (j = 0; j < 3; j++) { twoD[i][j] = k++; } } // print elements of 2D array for (i = 0; i < 3; i++) { for (j = 0; j < 3; j++) { System.out.print(twoD[i][j] + " "); } System.out.println(); } } }
Output of the above program
0 1 2 3 4 5 6 7 8
When you allocate memory for multi-dimensional array, you can specify the memory for the first (leftmost) dimension. You can allocate the remaining dimensions separately.
Example:
int twoD[][] = new int[3][]; twoD[0] = new int[4]; twoD[1] = new int[4]; twoD[2] = new int[4];
There is no advantage of individually allocating the second dimension in this situation.
Let's see an example with working program.
// demonstrate 2D array where manually allocate different size of second dimensions public class UnEqualTwoDArray { public static void main(String[] args) { int twoD[][] = new int[3][]; twoD[0] = new int[1]; twoD[1] = new int[2]; twoD[2] = new int[3]; for (int i = 0; i < twoD.length; i++) { for (int j = 0; j < twoD[i].length; j++) { twoD[i][j] = i * j; } } // print elements of twoD array for (int i = 0; i < twoD.length; i++) { for (int j = 0; j < twoD[i].length; j++) { System.out.print(twoD[i][j] + " "); } System.out.println(); } } }
Output:
0 0 1 0 2 4
Let's see one more example of multidimensional array. The following program creates 3 by 4 by 5, three dimensional array.
// Demonstrate a three-dimensional array. class ThreeDMatrix { public static void main(String args[]) { // 3D array of 3 by 4 by 5 int threeD[][][] = new int[3][4][5]; int i, j, k; for (i = 0; i < 3; i++) { for (j = 0; j < 4; j++) { for (k = 0; k < 5; k++) { threeD[i][j][k] = i * j * k; } } } // print 3D array for (i = 0; i < 3; i++) { for (j = 0; j < 4; j++) { for (k = 0; k < 5; k++) { System.out.print(threeD[i][j][k] + " "); } System.out.println(); } System.out.println(); } } }
Example:
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 2 3 4 0 2 4 6 8 0 3 6 9 12 0 0 0 0 0 0 2 4 6 8 0 4 8 12 16 0 6 12 18 24
Alternative Array Declaration Syntax
Declare one dimension array.
type[ ] var-name;
OR
type var-name[ ];
Example:
Following two declarations are equivalent:
int a1[] = new int[5]; int[] a2 = new int[5];
Declare multidimensional array.
type[ ][ ] var-name;
OR
type var-name[ ][ ];
Example:
Following two declarations are equivalent:
int a1[][] = new int[3][5]; int[][] a2 = new int[3][5];
You can declare multiple arrays at the same time using comma separated.
Example:
Following two declarations are equivalent:
int a1[], a2[], a3[]; // create three arrays of type int int[] b1, b2, b3; // create three arrays of type int
No comments :
Post a Comment