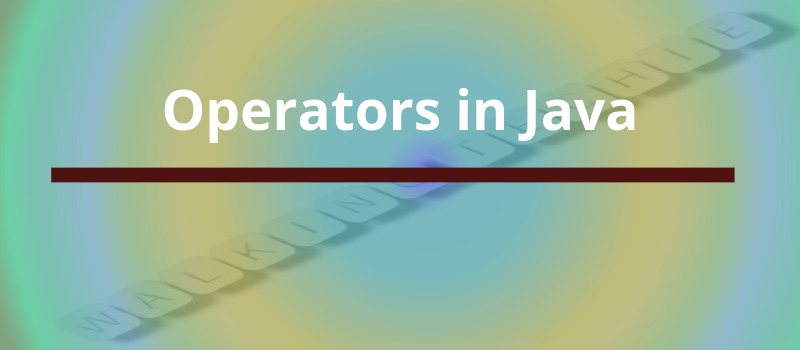
Operator in Java is symbol which is used to perform operation on operands.
Operand: Operand on which the operation is to be performed.
There are many types of operators in Java:
- Arithmetic Operator
- Bitwise Operator
- Relational Operator
- Boolean Logical Operator
- Ternary Operator
- Assignment Operator
Arithmetic Operator
Java arithmetic operations are used to perform mathematical expressions.
The operands of the arithmetic operators must be of a numeric type. You cannot use them on boolean types, but you can use them on char types, since the char type in Java is, essentially, a subset of int. Read more »
Bitwise Operator
Java defines several bitwise operators that can be applied to the integer types: long, int, short, char, and byte. These operators act upon the individual bits of their operands. Read more »
Relational Operator
The relational operators determine the relationship that one operand has to the other. Specifically, they determine equality and ordering.
Operator | Result |
---|---|
== | Equal to |
!= | Not equal to |
> | Greater than |
< | Less than |
>= | Greater than or equal to |
<= | Less than or equal to |
The outcome of these operations is a boolean value. Only integer, floating-point, and character operands may be compared to see which is greater or less than the other.
// Demonstrate the relational operators. public class RelationalOperator { public static void main(String[] args) { int x = 4, y = 4; boolean isEqual = x == y; // assign true to isEqual if (isEqual) { System.out.println("Both x and y are equal."); } isEqual = x != y; // assign false to isEqual System.out.println("Value of isEqual " + isEqual); x = 5; y = 12; boolean a = x > y; boolean b = x < y; boolean c = x >= y; boolean d = x <= y; System.out.println("Value of a = " + a); System.out.println("Value of b = " + b); System.out.println("Value of c = " + c); System.out.println("Value of d = " + d); } }
Output:
Both x and y are equal. Value of isEqual false Value of a = false Value of b = true Value of c = false Value of d = true
Boolean Logical Operator
The Boolean logical operators shown here operate only on boolean operands. All of the binary logical operators combine two boolean values to form a resultant boolean value.
Ternary Operator
Java ternary operator(?) is one line replacement for if-then-else statement. It is the only conditional operator which takes three operands. Its general form:
Here, if expression1 is true then expression2 will be evaluated otherwise expression3 will be evaluated.
// demonstrate ternary operator ? public class TernaryOperator { public static void main(String[] args) { int a = 5; int b = 10; int c = (a < b) ? a : b; System.out.println("c = " + c); boolean d = a < b ? true : false; System.out.println("a < b = " + d); } }
Assignment Operator
The assignment operator is the single equal sign, =. This operator has lowest precedence and give right-hand expression.
General form of assignment operator:
Here, the type of var must be compatible with the type of expression.
The assignment operator allow you to create chain of assignments. Consider below piece of code.
int x, y, z; x = y = z = 100; // set x, y, and z to 100
Precedence of the operators
Below table shows the order of precedence for Java operators, from highest to lowest. Operators in the same row are equal in precedence. In binary operations, the order of evaluation is left to right (except for assignment, which evaluates right to left).
Highest | ||||||
---|---|---|---|---|---|---|
++(postfix) | --(postfix) | |||||
++(prefix) | --(prefix) | ~ | ! | +(unary) | -(unary) | (type-cast) |
* | / | % | ||||
+ | - | |||||
>> | >>> | << | ||||
> | >= | < | <= | instanceof | ||
== | != | |||||
& | ||||||
^ | ||||||
| | ||||||
&& | ||||||
|| | ||||||
?: | ||||||
-> | ||||||
= | op= | |||||
Lowest |
No comments :
Post a Comment