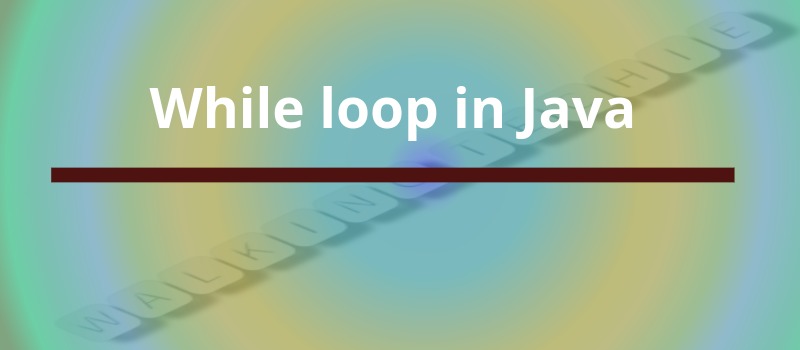
The Java while loop is a control flow statement that controls the execution of the programs repeatedly on the basis of given boolean condition.
Syntax:
while (condition) { // code to be executed }
The condition can be any boolean expression. The body of the loop will be executed as long
as the conditional expression is
true
. When condition becomes false
, control passes to the
next line of code immediately following the loop. The curly braces are unnecessary if only a
single statement is being repeated.
Flowchart:

Example:
// demonstrate the while loop public class WhileLoopDemo { public static void main(String[] args) { int i = 10; while (i >= 0) { System.out.println("i= " + i); i--; // decrease value of i by 1 } } }
Output:
i= 10 i= 9 i= 8 i= 7 i= 6 i= 5 i= 4 i= 3 i= 2 i= 1 i= 0
Java infinite while loop
If you pass true
or boolean expression that will be always true
after evaluation in the
while loop,
it will be infinite loop.
Syntax:
while (true) { // code to be executed }
Example:
// demonstrate the infinite while loop public class InfiniteWhileLoop { public static void main(String[] args) { int i = 0, j = 0; while (i == j) { // value of i and j will be always same System.out.println("Infinite while loop.."); // post increment operator i++; j++; } } }
Output:
Infinite while loop.. Infinite while loop.. Infinite while loop.. Infinite while loop.. Infinite while loop.. ctrl+c
Now, you need to press ctrl+c
to exit from the program.
Empty body of while loop
The body of the while (or any other of Java’s loops) can be empty. This is because a null statement (one that consists only of a semicolon) is syntactically valid in Java. For example, consider the following program:
/*Loop can have empty body. * Demonstrate the while loop with empty body*/ class NoBody { public static void main(String args[]) { int i, j; i = 100; j = 200; // find midpoint between i and j while (++i < --j) ; // no body in this loop System.out.println("Midpoint is " + i); } }
Output:
Midpoint is 150
No comments :
Post a Comment