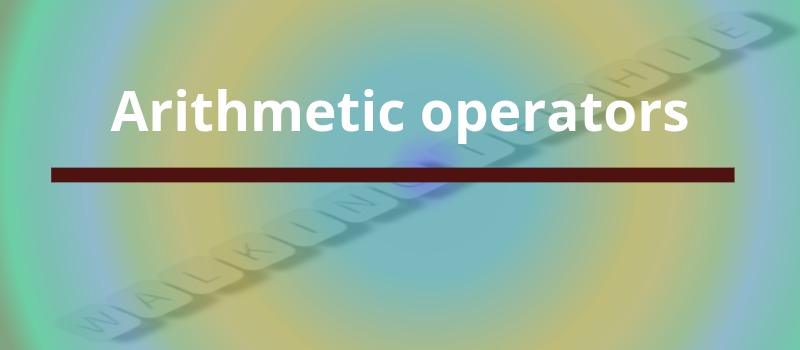
The operands of the arithmetic operators must be of a numeric type. You cannot use them on boolean types, but you can use them on char types, since the char type in Java is, essentially, a subset of int.
List of arithmetic operators:
Operator | Result |
---|---|
+ | Addition (also unary plus) |
- | Subtraction (also unary minus) |
* | Multiplication |
/ | Division |
% | Modulus |
++ | Increment |
+ = | Addition assignment |
-= | Subtraction assignment |
*= | Multiplication assignment |
/= | Division assignment |
%= | Modulus assignment |
-- | Decrement |
Basic arithmetic operation
The basic arithmetic operations like addition, subtraction, multiplication, and division perform the same way as we read in mathematics.
The unary minus operator negates its single operand.
The unary plus operator simply returns the value of its operand.
public class BasicMathOperator { public static void main(String[] args) { int x = 3, y = 2; // arithmetic using integers System.out.println("Integer Arithmetic"); int a = x + y; int b = x - y; int c = x * y; int d = x / y; int e = -x; // unary minus int f = +x; // unary plus System.out.println("a = " + a); System.out.println("b = " + b); System.out.println("c = " + c); System.out.println("d = " + d); System.out.println("e = " + e); System.out.println("f = " + f); // arithmetic using double System.out.println("\nFloating point Arithmetic"); double da = x + y; double db = x - y; double dc = x * y; double dd = x / y; double de = -x; // unary minus double df = +x; // unary plus System.out.println("da = " + da); System.out.println("db = " + db); System.out.println("dc = " + dc); System.out.println("dd = " + dd); System.out.println("de = " + de); System.out.println("df = " + df); } }
output:
Integer Arithmetic a = 5 b = 1 c = 6 d = 1 e = -3 f = -3 Floating point Arithmetic da = 5.0 db = 1.0 dc = 6.0 dd = 1.0 de = -3.0 df = 3.0
The Modulus Operator
The modulus operator, %, returns the remainder of a division operation. It can be applied to floating-point types as well as integer types.
// Demonstrate the % operator. class Modulus { public static void main(String args[]) { int x = 35; double y = 47.67; System.out.println("x mod 10 = " + x % 10); System.out.println("y mod 10 = " + y % 10); System.out.println("x mod 9.8 = " + x % 9.8); System.out.println("y mod 9.8 = " + y % 9.8); } }
output:
x mod 10 = 5 y mod 10 = 7.670000000000002 x mod 9.8 = 5.599999999999998 y mod 9.8 = 8.469999999999999
Arithmetic Compound Assignment Operators
You can combine arithmetic operator with an assignment.
Following are quite common in programming:
In Java, you can rewrite the satement as shown below:
This version uses the += compound assignment operator.
The compound assignment operators provide two benefits. First, they save you a bit of typing, because they are “shorthand” for their equivalent long forms. Second, in some cases they are more efficient than are their equivalent long forms.
Lets see an example of compound assignment operator.
// demonstrate compound assignment operator public class CompoundOperator { public static void main(String[] args) { int a = 1, b = 2; int c = 3, d = 4, e = 5; a += 10; b -= 4; c *= 3; d /= 6; e %= 3; System.out.println("a = " + a); System.out.println("b = " + b); System.out.println("c = " + c); System.out.println("d = " + d); System.out.println("e = " + e); } }
output:
a = 11 b = -2 c = 9 d = 0 e = 2
Increment and Decrement
The ++ and the – – are Java’s increment and decrement operators.
The increment operator increases its operand by one. The decrement operator decreases its operand by one.
The statement x = x + 1; can be rewritten using increment operator like below:
The statement x = x - 1; can be rewritten using decremet operator like below:
x++ and x-- is called postfix form. ++x and --x is called prefix form.
In the prefix form, the operand is incremented or decremented before the value is obtained for use in the expression.
In postfix form, the previous value is obtained for use in the expression, and then the operand is modified.
// Demonstrate prefix and postfix form. class IncDecDemo { public static void main(String args[]) { int a = 5; int b = 6; int c = a++; // c assign to 5 int d = ++b; // d assign to 7 System.out.println("Postfix form::"); System.out.println("c = " + c); System.out.println("d = " + d); System.out.println("a = " + a); System.out.println("b = " + b); a = 10; b = 11; int e = a--; // e assign to 10 int f = --b; // f assign to 10 System.out.println("\nPrefix form::"); System.out.println("e = " + e); System.out.println("f = " + f); System.out.println("a = " + a); System.out.println("b = " + b); } }
output:
Postfix form:: c = 5 d = 7 a = 6 b = 7 Prefix form:: e = 10 f = 10 a = 9 b = 10
This post is so helpfull and informative.Keep updating with more information...
ReplyDeleteFull Stack Course In Mumbai
Full Stack Course In Ahmedabad
Full Stack Course In Kochi
Full Stack Course In Trivandrum
Full Stack Development Course In Kolkata
More impressive Blog!!! Thanks for sharing this wonderful blog with us.
ReplyDeleteTableau Training in Chennai
Tableau Course in Chennai
Great Post.Thanks for sharing it with us. Keep up with your work.
ReplyDeleteJava Classes in Nagpur
This article on arithmetic operators in Java is a great resource! Clear explanations and examples make it easy to understand how these operators work. As someone brushing up on Java, I appreciate the straightforward breakdown. Now I am completing my java training course in Kolkata. Looking forward to applying these ideas to my coding projects!
ReplyDeleteThanks for the post. It was very interesting.
ReplyDeleteJava classes in Pune