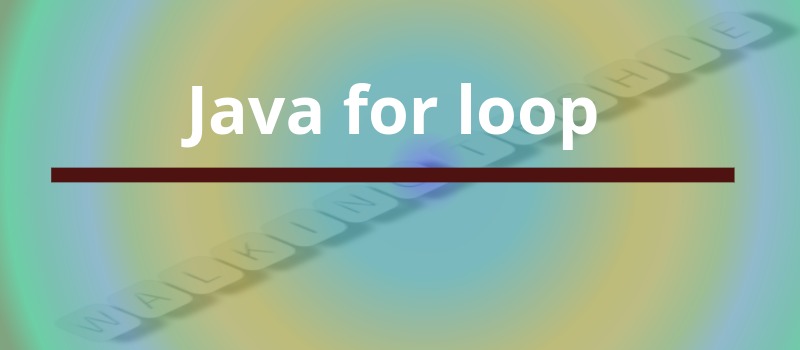
In this post, we will learn about for loop control statement. The Java for statement work much like the for loop statement in any other programming language.
Syntax:
- The initialization portion of for loop set loop control variable to initial value.
- The condition portion of for loop is boolean expression that tests the loop control variable.
If the outcome is
true
then the loop continues to iterate. If it isfalse
then the loop terminates. - The iteration expression determines how the loop control variable is changed each time the loop iterates.
Flowchart:

Example:
/* Demonstrate the for loop control statement in java. Call this file as ForDemo.java compile: javac ForDemo.java Run: java ForDemo */ public class ForDemo { public static void main(String[] args) { int i; // declare a variable i for (i = 0; i < 5; i++) System.out.println("The value of i:: " + i); } }When you run this program. output of this program shown below.
The value of i:: 0 The value of i:: 1 The value of i:: 2 The value of i:: 3 The value of i:: 4
In this example, i is the loop control variable. It is initialized to zero in the initialization portion of the for. At the start of each iteration (including the first one), the conditional test i < 5 is performed. If the outcome of this test is true, the println() statement is executed, and then the iteration portion of the loop is executed, which increases i by 1. This process continues until the conditional test is false.
The increment operator is ++, x++ which perform more efficiently than like x = x + 1;. The increment operator increases its operand by one.
Java also provides a decrement operator, which is specified as – –. This operator decreases its operand by one.
Declaring Loop Control Variables Inside the for Loop
Often the variable that controls a for loop is needed only for the purposes of the loop and is not used elsewhere. In such case, it is possible to declare the variable inside the initialization portion of for loop.
Example:
// Test a given number is prime class FindPrime { public static void main(String args[]) { int num; boolean isPrime; num = 17; if (num < 2) isPrime = false; else isPrime = true; for (int i = 2; i <= num / i; i++) { if ((num % i) == 0) { isPrime = false; break; } } if (isPrime) System.out.println(num + " is a prime number."); else System.out.println(num + " is not a prime number."); } }
Output:
17 is a prime number.
When you declare a variable inside the for loop, then the scope of the variable limited to it. If you need to use the loop control variable somewhere in the program, then you will not be able to declare it inside the for loop.
Declare multiple loop control variables using comma
Java provides flexibility to declare multiple loop control variable inside the initialization portion of the for loop. Each variable is separated from next by a comma. Even you can also include multiple statement inside iteration portion.
Example:
// Demonstrate multiple loop control variable declaration and initialization // We can have multiple statement inside iteration portion public class CommaSeparated { public static void main(String[] args) { for (int a = 1, b = 5; a < b; a++, b--) { System.out.println("a = " + a); System.out.println("b = " + b); } } }
Output:
a = 1 b = 5 a = 2 b = 4
Java infinite for loop
If you use two semicolons ;; in the for loop, it will be infinite for loop.
Syntax:
for ( ; ; ) { // code to be executed }
Example 1:
// demonstrate infinite for loop public class InfiniteForLoop { public static void main(String[] args) { for (; ; ) { System.out.println("Infinite for loop.."); } } }
Example 2:
// demonstrate infinite for loop public class InfiniteForDemo { public static void main(String[] args) { boolean flag = false; for (; !flag; ) { System.out.println("Infinite for loop.."); } } }
Output:
Infinite while loop.. Infinite while loop.. Infinite while loop.. Infinite while loop.. Infinite while loop.. ctrl+c
Now, you need to press ctrl+c
to exit from the program.
The for-each version of the for loop
The for-each version of for loop is introduced from the beginning with JDK 5. The for-each style of for is also referred to as the enhanced for loop. The for-each loop is used to traverse the collection or array in Java. It is easier to use than simple for loop because we don't need to increment value and use subscript notation.
Syntax:
for(type itr-var : collection) { // code to be executed }
- type specifies the type.
- itr-var specifies the name of an iteration variable that will receive the elements from a collection, one at a time, from beginning to end.
- With each iteration of the loop, the next element in the collection is retrieved and stored in itr-var. The loop repeats until all elements in the collection have been obtained.
- type must be the same as (or compatible with) the elements stored in the collection.
Example:
// demonstrate for-each version of the for loop public class ForEachExample { public static void main(String[] args) { int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int sum = 0; for (int x : arr) { System.out.println("Value of x: " + x); sum += x; } System.out.println("Sum of value from 1 to 10 is: " + sum); } }
Output:
Value of x: 1 Value of x: 2 Value of x: 3 Value of x: 4 Value of x: 5 Value of x: 6 Value of x: 7 Value of x: 8 Value of x: 9 Value of x: 10 Sum of value from 1 to 10 is: 55
As this output shows, the for-each style for automatically cycles through an array in sequence from the lowest index to the highest.
Important point: The for-each style for loop iteration variable is "read only" as it relates to the underlying array. An assignment to the iteration variable has no effect on the underlying array.
Example:
// The for-each loop is essentially read-only. public class ForEachNoChange { public static void main(String[] args) { int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; for (int x : arr) { System.out.print(x + " "); x = x * 2; // no effects on arr } System.out.println(); for (int x : arr) { System.out.print(x + " "); } } }
Output:
1 2 3 4 5 6 7 8 9 10 1 2 3 4 5 6 7 8 9 10
Nested for loop
Java allows loops to be nested.
Example:
// Nested for loop example public class NestedForLoop { public static void main(String[] args) { for (int i = 1; i <= 5; i++) { for (int j = i; j <= 5; j++) { System.out.print(j + " "); } System.out.println(); } } }
Output:
1 2 3 4 5 2 3 4 5 3 4 5 4 5 5
No comments :
Post a Comment