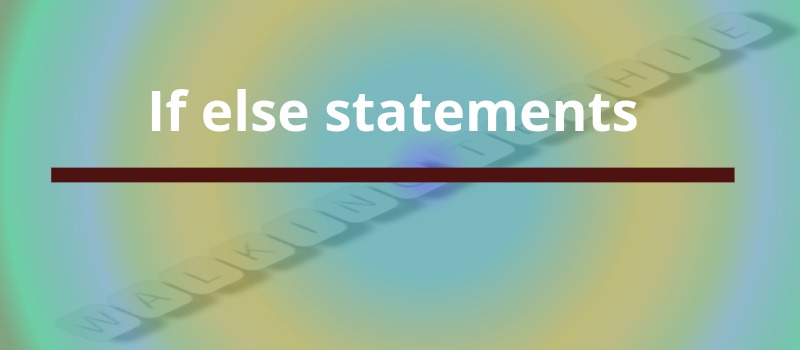
Java if statement is used to control the flow of program's execution based upon conditions. Condition evaluated to either true or false. There are various type of if statements in Java:
- if statement
- if-else statement
- if-else-if ladder
- nested if statement
Java if statement
The if statement is Java’s conditional branch statement. It can be used to route program execution through two different paths.
Java if statement test the condition. It execute the if statement or if block only if condition is true. If condition is false then statement or block of statements is bypassed. Here is the general form of the if statement:
if (condition) statement;OR
if (condition) { //..... //code to be executed //..... }
You can see an example of if(condition) statement.
// demonstrate If statement public class IfDemo { public static void main(String[] args) { // declare a num variable and initialise 20 to it int num = 20; // relation expression // evaluate to true or false if (num > 10) { System.out.println("Num is greater than 10."); } } }
Output:
Num is greater than 10.p>We have seen the expression used to control if statement is relational operators. It can control using the boolean variable. Example:
// Demonstrate if statement using boolean variable public class IfBooleanDemo { public static void main(String[] args) { boolean flag = true; int a = 5; if (flag) System.out.println("This is an example of single statement."); if (flag) { a = 10; a = a >> 2; System.out.println("\nThis is an example of block of statements."); System.out.println("Value of a = " + a); } } }
Output:
This is an example of single statement. This is an example of block of statements. Value of a = 2
Java if statement
Java if statement test the condition. It execute the if statement or if block only if condition is true. If condition is false then else statement or block of statements is executed. Here is the general form of the if-else statement:
if (condition) statement1; else statement2;OR
if (condition) { //..... //code to be executed //..... }else { // ....... // else code to be executed // ...... }
// demonstrate if-else statement public class IfElseDemo { public static void main(String[] args) { int num = 12; // single if-else statement if (num % 2 == 0) System.out.println("num is even."); else System.out.println("num is odd."); num = 21; // single if and block of else statements if (num % 2 == 0) System.out.println("\nnum is even."); else { System.out.println("\nBlock of statements in else block."); System.out.println("num is odd."); } // best practice to write statement's in opening curly and curly braces if (num >= 21) { System.out.println("\nnum is greater than or equal to 21"); } else { System.out.println("\nnum is less than 21"); } } }
Output:
num is even. Block of statements in else block. num is odd. num is greater than or equal to 21
if-else-if ladder
The if-else-if ladder statement executes one of the condition from the multiple statements.
if (condition1) { //code to be executed if condition1 is true } else if (condition2) { //code to be executed if condition2 is true } else if (condition3) { //code to be executed if condition3 is true } ... else { //code to be executed if all the conditions are false }
The if statements are executed from the top down. As soon as one of the if conditions is true then the corresponding associated if statements is executed, and rest of the ladder is bypassed. If none of the conditions is true, then the final else statement will be executed. The else act as default condition; it will be executed if all other conditional tests fail. If there is no final else and all other conditions are false, then no action will take place.
// Demonstrate if-else-if statements. class IfElseIfLadder { public static void main(String args[]) { int month = 4; // April String season; if (month == 12 || month == 1 || month == 2) season = "Winter"; else if (month == 3 || month == 4 || month == 5) season = "Spring"; else if (month == 6 || month == 7 || month == 8) season = "Summer"; else if (month == 9 || month == 10 || month == 11) season = "Autumn"; else season = "Bogus Month"; System.out.println("April is in the " + season + "."); } }
Output:
April is in the Spring.
nested if statement
The nested if statement represents the if block within another if block or else block.
if (condition) { // code to be executed if (condition) { // code to be executed } } else { if (condition) { // code to be executed } }
// Demonstrate Nested if public class NestedIfDemo { public static void main(String[] args) { int i = 10, j = 30; int a = 0, b = -1; int k = 120; int c = 12, d = 21; if (i == 10) { if (j < 20) a = b; if (k > 100) c = d; // this if is else a = c; // associated with this else } else a = d; // this else refers to if(i == 10) System.out.println("Value of i = " + i + " j = " + j); System.out.println("Value of a = " + a + " b= " + b); System.out.println("Value of c = " + c + " d= " + d); System.out.println("Value of k = " + k); } }
Output:
Value of i = 10 j = 30 Value of a = 0 b= -1 Value of c = 21 d= 21 Value of k = 120
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
ReplyDeletefactorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.