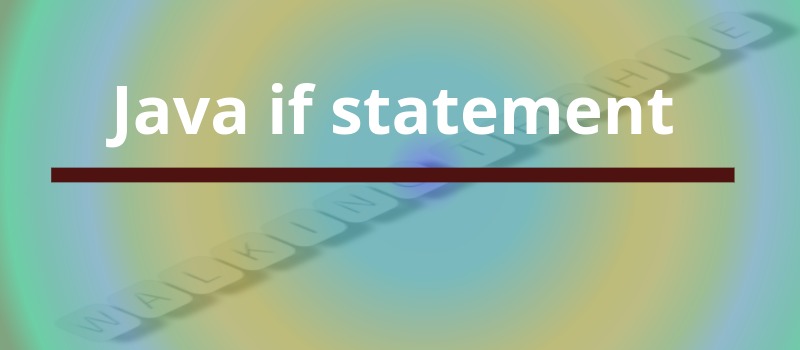
In this post, we will learn about if control statement. The Java if statement work much like the IF statement in any other programming language. The simplest syntax of if statement:
if(condition) statement;
Here, condition is a Boolean expression. If condition is true, then the statement is executed. If condition is false, then the statement is bypassed.
if(num < 10) System.out.println("num is less than 10");
In this case, if num contains a value that is less than 10, the conditional expression is true, and println( ) will execute. If num contains a value greater than or equal to 10, then the println( ) method is bypassed.
some of relational operatorsOperator | Meaning |
---|---|
< | Less than |
> | Greater than |
== | Equal |
/* Demonstrate the if statement in java. Call this file as IfDemo.java compile: javac IfDemo.java Run: java IfDemo */ class IfDemo { public static void main(String[] args) { int x, y; // declare two variables x and y x = 10; // assign 10 to x y = 20; // assign 20 to y if (x < y) System.out.println("x is less than y"); x = x * 2; if (x == y) System.out.println("x now equal to y"); x = x * 2; if (x > y) System.out.println("x now greater than y"); // false statement. this won't display on terminal if (x == y) System.out.println("You won't see this"); } }When you run this program. output of this program shown below.
x is less than y x now equal to y x now greater than y
No comments :
Post a Comment