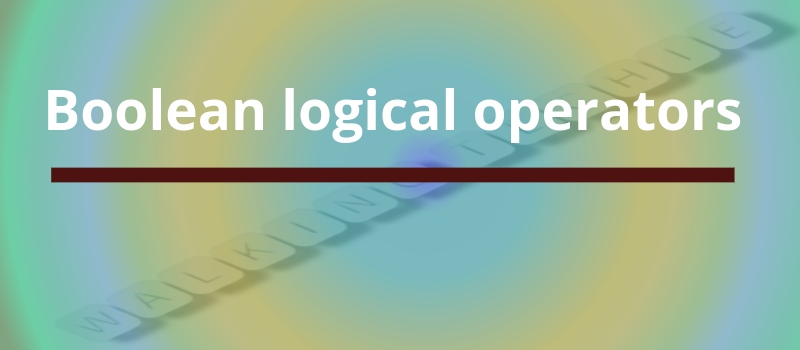
The Boolean logical operators shown here operate only on boolean operands. All of the binary logical operators combine two boolean values to form a resultant boolean value.
Operator | Result |
---|---|
& | Logical AND |
| | Logical OR |
^ | Logical XOR (exclusive OR) |
|| | Short-circuit OR |
&& | Short-circuit AND |
! | Logical unary NOT |
&= | AND assignment |
|= | OR assignment |
^= | XOR assignment |
== | Equal to |
!= | Not equal to |
?: | Ternary if-then-else |
The logical Boolean operators, &, |, and ^, operate on boolean values in the same way that they operate on the bits of an integer. The logical ! operator inverts the Boolean state: !true == false and !false == true.
A | B | A | B | A & B | A ^ B | !A |
---|---|---|---|---|---|
false | false | false | false | false | true |
false | true | true | false | true | true |
true | false | true | false | true | false |
true | true | true | true | false | false |
Here is the program which is almost same as BitwiseLogical.
// Demonstrate the boolean logical operators. public class BooleanLogical { public static void main(String[] args) { boolean a = true; boolean b = false; boolean c = a | b; boolean d = a & b; boolean e = a ^ b; boolean f = (!a & b) | (a & !b); boolean g = !a; System.out.println(" a = " + a); System.out.println(" b = " + b); System.out.println(" a|b = " + c); System.out.println(" a&b = " + d); System.out.println(" a^b = " + e); System.out.println("!a&b|a&!b = " + f); System.out.println(" !a = " + g); } }
Output:
a = true b = false a|b = true a&b = false a^b = true !a&b|a&!b = true !a = false
Short-Circuit Logical Operators
The && and || is called as short-circuit logical operator. The logical OR operator results in true when A is true, no matter what B is. Similarly, the logical AND operator results in false when A is false, no matter what B is.
If you use the || and && forms, rather than the | and & forms of these operators, Java will not bother to evaluate the right-hand operand when the outcome of the expression can be determined by the left operand alone.
// demonstrate short circuit logical operator public class ShortCircuitOperator { public static void main(String[] args) { int a = 0; int num = 100; if (a != 0 && num / a > 4) { System.out.println("It won't print."); } a = 3; if (a != 0 && num / a > 4) { System.out.println("It will print."); } } }
Output:
It will print.
Lets reuse the same above program. Now use the single & version of AND, both sides would be evaluated, causing a run-time exception when a is zero.
// demonstrate logical AND operator exception case public class LogicalAndExceptionDemo { public static void main(String[] args) { int a = 0; int num = 100; if (a != 0 & num / a > 4) { System.out.println("It throw ArithmeticException"); } } }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero at LogicalAndExceptionDemo.main(LogicalAndExceptionDemo.java:6)
It is standard practice to use the short-circuit forms of AND and OR in cases involving Boolean logic, leaving the single-character versions exclusively for bitwise operations.
There are exceptions to this rule. For example, consider the following statement:
if(c==1 & e++ < 100) d = 100;
Here, using a single & ensures that the increment operation will be applied to e whether c is equal to 1 or not.
No comments :
Post a Comment