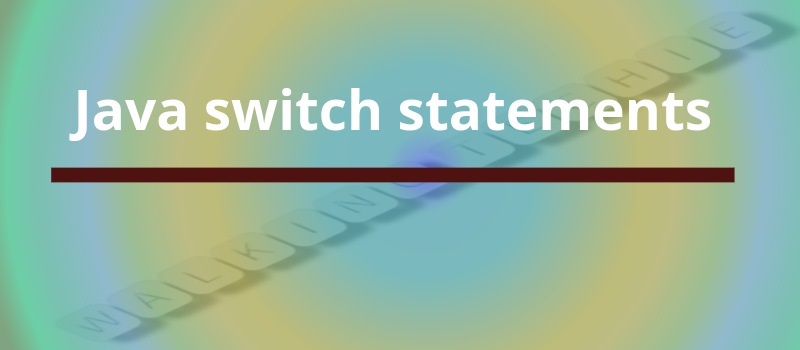
The switch statement is Java’s multiway branch statement. It provides an easy way to dispatch execution to different parts of your code based on the value of an expression. Here is general form of switch statement:
switch (expression) { case value1: // statement sequence break; // optional case value2: // statement sequence break; // optional ...... ...... ...... case valueN : // statement sequence break; // optional default: // default statement sequence }
Switch provides better alternative than a large series of if-else-if statements. Prior to JDK 7, expression must be of type byte, short, int, char, Byte, Short, Integer, Character or enum. Beginning with JDK 7, expression can also be of type String.
Important point to be noted:
- There can be one or N number of case values for a switch expression.
- Each value specified in case statement must be unique. In case of duplicate value, it renders compile-time error.
- Type of each value must be compatible with the type of expression. The value of case must be unique constant expression or literal.
- The Java switch expression must be of byte, short, int, char (with its Wrapper type), enums and string.
- Each case statement can have a break statement which is optional. When control reaches to the break statement, it jumps the control after the switch expression. If a break statement is not found, it executes all the subsequent cases.
- If none of the constants matches the value of the expression, then the default statement is executed.
- Switch can have optional default statement.
- If no case matches and no default is present, then no further action is taken.
- The break statement is used inside the switch to terminate a statement sequence.
// demonstration of Switch public class SwitchDemo { public static void main(String[] args) { // variable for switch expression int exp = 10; switch (exp) { case 5: System.out.println("5"); break; case 10: System.out.println("10"); break; case 15: System.out.println("15"); break; default: // default case statement System.out.println("Not in 5, 10 or 15"); } } }
Output:
10
Lets see another example of switch statement inside for loop.
// A simple example of the switch. class SampleSwitch { public static void main(String args[]) { for (int i = 0; i < 6; i++) switch (i) { case 0: System.out.println("i is zero."); break; case 1: System.out.println("i is one."); break; case 2: System.out.println("i is two."); break; case 3: System.out.println("i is three."); break; default: System.out.println("i is greater than 3."); } } }
Output:
i is zero. i is one. i is two. i is three. i is greater than 3. i is greater than 3.
Java switch statement is fall-through
The break statement is optional. If you omit the break, execution will continue on into the next case. It is sometimes desirable to have multiple cases without break statements between them.
// In a switch, break statements are optional. class MissingBreak { public static void main(String args[]) { for (int i = 0; i < 12; i++) switch (i) { case 0: case 1: case 2: case 3: case 4: System.out.println("i is less than 5"); break; case 5: case 6: case 7: case 8: case 9: System.out.println("i is less than 10"); break; default: System.out.println("i is 10 or more"); } } }
Output:
i is less than 5 i is less than 5 i is less than 5 i is less than 5 i is less than 5 i is less than 10 i is less than 10 i is less than 10 i is less than 10 i is less than 10 i is 10 or more i is 10 or more
Lets see more practical example
public class SeasonSwitch { public static void main(String args[]) { int month = 4; String season; switch (month) { case 12: case 1: case 2: season = "Winter"; break; case 3: case 4: case 5: season = "Spring"; break; case 6: case 7: case 8: season = "Summer"; break; case 9: case 10: case 11: season = "Autumn"; break; default: season = "Bogus Month"; } System.out.println("April is in the " + season + "."); } }
Output:
April is in the Spring.
public class SwitchFallThrough { public static void main(String[] args) { int exp = 10; switch (exp) { case 5: System.out.println("5"); case 10: System.out.println("10"); case 15: System.out.println("15"); case 20: System.out.println("20"); case 25: System.out.println("25"); default: // default case statement System.out.println("Default statement."); } } }
Output:
10 15 20 25 Default statement.
Java switch statement with String
Beginning with JDK 7, you can use String to control a switch statement.
class StringSwitch { public static void main(String args[]) { String str = "two"; switch (str) { case "one": System.out.println("one"); break; case "two": System.out.println("two"); break; case "three": System.out.println("three"); break; default: System.out.println("no match"); break; } } }
Output:
two
Switching on strings can be more expensive than switching on integers. Therefore, it is best to switch on strings only in cases in which the controlling data is already in string form. In other words, don’t use strings in a switch unnecessarily.
Java Nested Switch Statement
You can use switch statement inside other switch statement in Java. It is known as nested switch statement. Since a switch statement defines its own block, no conflicts arise between the case constants in the inner switch and those in the outer switch.
public class NestedSwitch { public static void main(String[] args) { int exp = 1, target = 0; switch (exp) { case 1: switch (target) { // nested switch case 0: System.out.println("Inner switch exp: 1"); System.out.println("target is zero"); break; case 1: // no conflicts with outer switch System.out.println("target is one"); break; } System.out.println("Outer switch exp: 1"); break; case 2: target = 3; System.out.println("Outer switch: 2"); break; } } }
Output:
Inner switch exp: 1 target is zero Outer switch exp: 1
In summary, there are three important features of the switch statement to note:
- The switch differs from the if in that switch can only test for equality, whereas if can evaluate any type of Boolean expression. That is, the switch looks only for a match between the value of the expression and one of its case constants.
- No two case constants in the same switch can have identical values. Of course, a switch statement and an enclosing outer switch can have case constants in common.
- A switch statement is usually more efficient than a set of nested ifs.
Java using enum with switch statement
Java allow to use enum with switch statement. Lets see an example:
public class EnumSwitchDemo { public enum Days { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY } public static void main(String[] args) { Days[] days = Days.values(); for (Days day : days) { switch (day) { case MONDAY: System.out.println("Monday"); break; case TUESDAY: System.out.println("Tuesday"); break; case WEDNESDAY: System.out.println("Wednesday"); break; case THURSDAY: System.out.println("Thursday"); break; case FRIDAY: System.out.println("friday"); break; case SATURDAY: System.out.println("Saturday"); break; case SUNDAY: System.out.println("Sunday"); break; } } } }
Output:
Sunday Monday Tuesday Wednesday Thursday friday Saturday
Java using wrapper with switch statement
Java allows to use four wrapper classes with swith statement. Wrapper classes are Character, Byte, Short, and Integer.
public class WrapperSwitchDemo { public static void main(String[] args) { // example of Character wrapper class Character exp = new Character('a'); switch (exp) { case 'a': System.out.println("a"); break; case 'b': System.out.println("b"); break; default: System.out.println("Other character."); } // example of Byte wrapper class with switch statement Byte age = new Byte((byte) 18); switch (age) { case 16: System.out.println("You are under 18."); break; case 18: System.out.println("You are eligible for vote."); break; case 65: System.out.println("You are senior citizen."); break; default: System.out.println("Please give the valid age."); break; } // example of Short wrapper class with switch statement Short age1 = new Short((short) 65); switch (age1) { case 16: System.out.println("You are under 18."); break; case 18: System.out.println("You are eligible for vote."); break; case 65: System.out.println("You are senior citizen."); break; default: System.out.println("Please give the valid age."); break; } // example of Integer wrapper class with switch statement Integer age2 = new Integer(16); switch (age2) { case 16: System.out.println("You are under 18."); break; case 18: System.out.println("You are eligible for vote."); break; case 65: System.out.println("You are senior citizen."); break; default: System.out.println("Please give the valid age."); break; } } }
Output:
a You are eligible for vote. You are senior citizen. You are under 18.
How Java compiler works with switch statement
When it compiles a switch statement, the Java compiler will inspect each of the case constants and create a "jump table" that it will use for selecting the path of execution depending on the value of the expression. Therefore, if you need to select among a large group of values, a switch statement will run much faster than the equivalent logic coded using a sequence of if-elses. The compiler can do this because it knows that the case constants are all the same type and simply must be compared for equality with the switch expression. The compiler has no such knowledge of a long list of if expressions.
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
ReplyDeletefactorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.