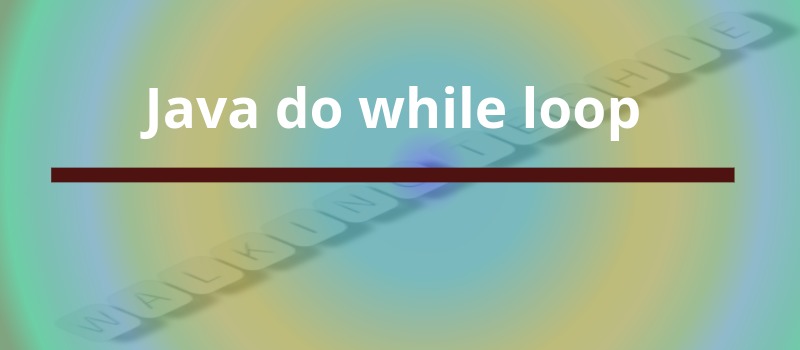
As you saw in Java
while loop, if the condition expression in while loop is
false
initially, then the body of the loop will not be executed at all. However, sometimes it is
desirable to execute the body of a loop at least once, even if the conditional expression is false
to begin with.
The Java do while loop is a control flow statement that executes the program at least once and the further execution depends on given boolean condition.
Syntax:
do { // code to be executed } while (condition);
Each iteration of the do-while loop first executes the body of the loop and then evaluates
the conditional expression. If this expression is true
, the loop will repeat. Otherwise, the
loop terminates. As with all of Java’s loops, condition must be a Boolean expression.
Flowchart:

Example:
// demonstrate the do-while loop public class DoWhileLoopDemo { public static void main(String[] args) { int i = 10; do { System.out.println("i= " + i); i--; // decrease value of i by 1 } while (i >= 0); } }
You can write above program more efficiently as below:
// demonstrate the do-while loop public class DoWhileLoopDemo { public static void main(String[] args) { int i = 10; do { System.out.println("i= " + i); } while (--i >= 0); } }
In this example, the expression (--i > 0) combines the decrement of i and the test for zero into one expression. Here is how it works. First, the --i statement executes, decrementing i and returning the new value of i. This value is then compared with zero. If it is greater than or equal to zero, the loop continues; otherwise, it terminates.
Output:
i= 10 i= 9 i= 8 i= 7 i= 6 i= 5 i= 4 i= 3 i= 2 i= 1 i= 0
Java infinite do-while loop
If you pass true
or boolean expression that will be always true
after evaluation in the
do-while loop, it will be infinite loop.
Syntax:
do { // code to be executed } while (true);
Example:
// demonstrate the infinite do-while loop public class InfiniteDoWhileLoop { public static void main(String[] args) { int i = 0, j = 0; do { System.out.println("Infinite while loop.."); // post increment operator i++; j++; } while (i == j); // value of i and j will be always same } }
Output:
Infinite while loop.. Infinite while loop.. Infinite while loop.. Infinite while loop.. Infinite while loop.. ctrl+c
Now, you need to press ctrl+c
to exit from the program.
The do-while loop is especially useful when you process a menu selection, because you will usually want the body of a menu loop to execute at least once.
Example:
// Using a do-while to process a menu selection class Menu { public static void main(String args[]) throws java.io.IOException { char choice; do { System.out.println("Enter either 1, 2, 3, 4 or 5 for help:"); System.out.println(" 1. if"); System.out.println(" 2. switch"); System.out.println(" 3. while"); System.out.println(" 4. do-while"); System.out.println(" 5. for\n"); System.out.println("Choose one:"); choice = (char) System.in.read(); } while (choice < '1' || choice > '5'); System.out.println("\n"); switch (choice) { case '1': System.out.println("The if:\n"); System.out.println("if(condition) statement;"); System.out.println("else statement;"); break; case '2': System.out.println("The switch:\n"); System.out.println("switch(expression) {"); System.out.println(" case constant:"); System.out.println(" statement sequence"); System.out.println(" break;"); System.out.println(" //..."); System.out.println("}"); break; case '3': System.out.println("The while:\n"); System.out.println("while(condition) statement;"); break; case '4': System.out.println("The do-while:\n"); System.out.println("do {"); System.out.println(" statement;"); System.out.println("} while (condition);"); break; case '5': System.out.println("The for:\n"); System.out.print("for(init; condition; iteration)"); System.out.println(" statement;"); break; } } }
Output:
Enter either 1, 2, 3, 4 or 5 for help: 1. if 2. switch 3. while 4. do-while 5. for Choose one: 2 The switch: switch(expression) { case constant: statement sequence break; //... }
In the above program, System.in.read( ) is used to read character from keyboard. System.in.read( ) is used here to obtain the user’s choice. It reads characters from standard input (returned as integers, which is why the return value was cast to char). By default, standard input is line buffered, so you must press enter before any characters that you type will be sent to your program.
One other point to consider: Because System.in.read( ) is being used, the program must specify the throws java.io.IOException clause.
No comments :
Post a Comment