What is Stack in Java?
Stack is an legacy class, available from JDK 1.0. Stack class is a subclass of Vector that implements a standard last-in, first-out(LIFO) stack. Java Stack only defines the default constructor, which creates an empty stack.
Stack extends class Vector with five operations that allow a vector to be treated as a stack. The usual push and pop operations are provided, as well as a method to peek at the top item on the stack, a method to test for whether the stack is empty, and a method to search the stack for an item and discover how far it is from the top.
Here is the topic that will cover in this post.
Java Stack class Diagram
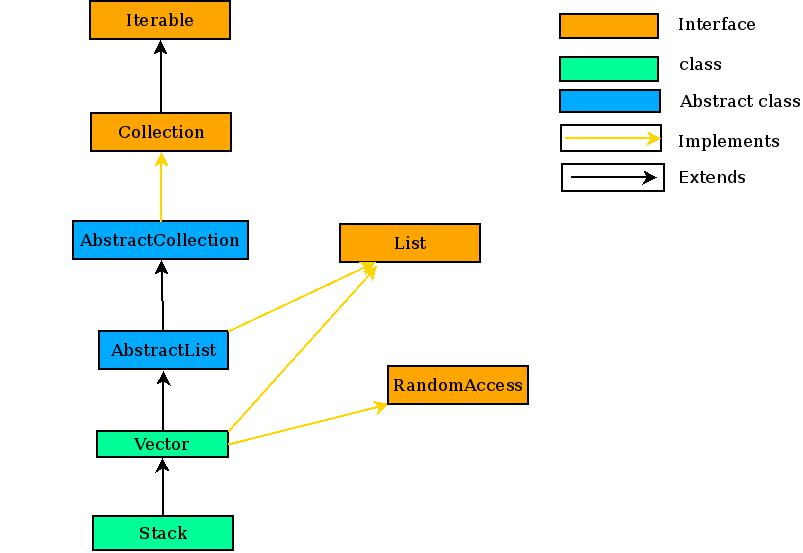
Java Stack class Declaration
Java Stack extends Vector class directly and implements RandomAccess, List, Collections etc interface indirectly. It is a LIFO list.
public class Stack<E> extends Vector<E>
Here, E specifies the type of element stored in the stack.
Java Stack features
- Java Stack inherits
Vector
class.- Java Stack permits
null
elements.- Java Stack internally uses dynamic array to store elements.
- Java Stack maintain an insertion order of elements.
- The iterators returned by this class's iterator() iterator and listIterator(int) listIterator methods are fail-fast
- The Enumeration Enumerations returned by elements() elements method are not fail-fast.
- Java Stack is synchronized legacy class.
- Java Stack available from JDK 1.0.
Java Stack constructors
Stack
has only one default constructor, which create an empty stack.
public Stack() { }
Java Stack methods
Stack includes all the methods defined by Vector and adds several of its own, shown in below table:
Method | Description |
---|---|
public boolean empty() | Returns true if the stack is empty, and returns false if the stack contains elements. |
public E peek() | Returns the element on the top of the stack, but does not remove it. |
public E pop() | Removes the object at the top of this stack and returns that object as the value of this function. |
public E push(E element) | Pushes element onto the top of this stack. element is also returned. |
public int search(Object element) | Searches for element in the stack. If found, its offset from the top of the stack is returned. Otherwise, –1 is returned. |
Example
Here is a simple program of Stack.
package com.walking.techie; import java.util.Stack; public class StackDemo { static void showPush(Stack<Integer> stack, int item) { stack.push(item); System.out.println("push(" + item + ")"); System.out.println("stack: " + stack); } static void showPop(Stack<Integer> stack) { System.out.print("pop -> "); Integer item = stack.pop(); System.out.println(item); System.out.println("stack: " + stack); } public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); System.out.println("Empty stack : " + stack); System.out.println("Empty stack : " + stack.isEmpty()); showPush(stack, 10); showPush(stack, 20); showPush(stack, 30); showPush(stack, 40); System.out.println("Non Empty stack : peek call : " + stack.peek()); System.out.println("Non Empty stack : peek call : search call : " + stack.search(40)); System.out.println("Non Empty stack : peek call : search call : " + stack.search(20)); showPop(stack); showPop(stack); showPop(stack); System.out.println("Empty stack : " + stack.isEmpty()); } }
Output of above program is shown below:
Empty stack : [] Empty stack : true push(10) stack: [10] push(20) stack: [10, 20] push(30) stack: [10, 20, 30] push(40) stack: [10, 20, 30, 40] Non Empty stack : peek call : 40 Non Empty stack : peek call : search call : 1 Non Empty stack : peek call : search call : 3 pop -> 40 stack: [10, 20, 30] pop -> 30 stack: [10, 20] pop -> 20 stack: [10] Empty stack : false
How Stack’s push() and pop() operations works Internally?
stack's push() and pop() are most frequently used operations. The push() operation used to insert an element at the top of the stack and pop() operation remove (pop) element from the top of the stack.
Java Stack data structure has one internal property called elementCount (like top) to refer top empty element of that stack. If Stack is empty, this elementCount refers to the first empty element. If we push an element in stack, it will insert an element to elementCount position in stack then increment elementCount by 1. When we pop an element from stack, first it will decrement elementCount by 1, then remove element from elementCount position. Empty Java Stack shown in below diagram:
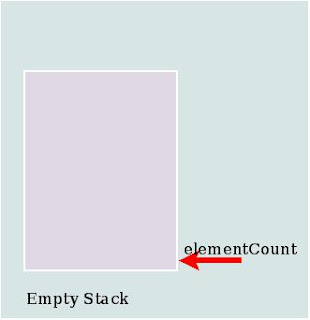
As shown in the below diagram, Stack’s Push operation always inserts new element at the elementCount position of the Stack.
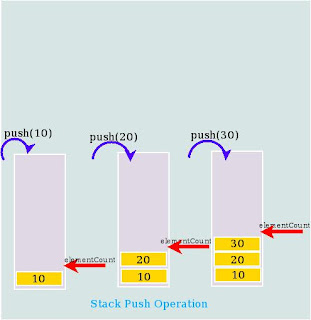
As shown in the below diagram, Stack’s Pop operation always removes an element from the elementCount-1 position of the Stack.
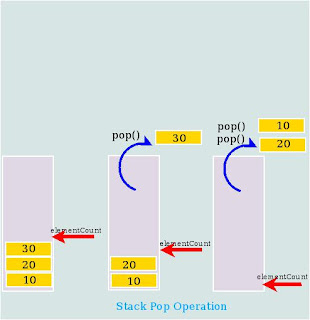
Iterate or Traverse over Stack
1. Iterating Stack in java using Java 8 forEach and lambda expression
package com.walking.techie; import java.util.Stack; public class StackTraversal { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(10); stack.push(20); stack.push(30); stack.push(40); stack.push(50); // using java 8 for each and lambda expression System.out.println("Stack element from bottom to top ---> : "); stack.forEach(e -> { System.out.print(e + " "); }); } }
Output of above program is shown below:
Stack element from bottom to top ---> : 10 20 30 40 50
2. Iterating Stack in java using Iterator
package com.walking.techie; import java.util.Iterator; import java.util.Stack; public class StackTraversal { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(10); stack.push(20); stack.push(30); stack.push(40); stack.push(50); Iterator<Integer> iterator = stack.iterator(); System.out.println("Stack element from bottom to top ---> : "); while (iterator.hasNext()) { System.out.print(iterator.next() + " "); } } }
Output of above program is shown below:
Stack element from bottom to top ---> : 10 20 30 40 50
3. Iterating Stack in java using Enumeration
package com.walking.techie; import java.util.Enumeration; import java.util.Stack; public class StackTraversal { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(10); stack.push(20); stack.push(30); stack.push(40); stack.push(50); Enumeration<Integer> enumeration = stack.elements(); System.out.println("Stack element from bottom to top ---> : "); while (enumeration.hasMoreElements()) { System.out.print(enumeration.nextElement() + " "); } } }
Output of above program is shown below:
Stack element from bottom to top ---> : 10 20 30 40 50
4. Iterating Stack in java using ListIterator
package com.walking.techie; import java.util.ListIterator; import java.util.Stack; public class StackTraversal { public static void main(String[] args) { Stack<String> stack = new Stack<>(); stack.push("Red"); stack.push("Blue"); stack.push("Green"); stack.push("White"); stack.push("Black"); ListIterator<String> stringIterator = stack.listIterator(); System.out.println("Stack element from bottom to top ---> : "); while (stringIterator.hasNext()) { System.out.print(stringIterator.next() + " "); } System.out.println(); System.out.println("\nStack element from top to bottom <--- : "); while (stringIterator.hasPrevious()) { System.out.print(stringIterator.previous() + " "); } stringIterator = stack.listIterator(stack.size()); System.out.println(); System.out.println("\nStack element from top to bottom <--- : "); while (stringIterator.hasPrevious()) { System.out.print(stringIterator.previous() + " "); } System.out.println(); System.out.println("\nStack element from bottom to top ---> : "); while (stringIterator.hasNext()) { System.out.print(stringIterator.next() + " "); } } }
Output of above program is shown below:
Stack element from bottom to top ---> : Red Blue Green White Black Stack element from top to bottom <--- : Black White Green Blue Red Stack element from top to bottom <--- : Black White Green Blue Red Stack element from bottom to top ---> : Red Blue Green White Black
5. Iterating Stack in java using for each loop
package com.walking.techie; import java.util.Stack; public class StackTraversal { public static void main(String[] args) { Stack<String> stack = new Stack<>(); stack.push("Red"); stack.push("Blue"); stack.push("Green"); stack.push("White"); stack.push("Black"); // Iterate using the for each on stack System.out.println("Stack element from bottom to top ---> : "); for (String string : stack) { System.out.print(string + " "); } } }
Output of above program is shown below:
Stack element from bottom to top ---> : Red Blue Green White Black
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
ReplyDeletefactorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
vegamovies Earlier people were not so much means to watch movies and to watch movies, we had to go to the movies hall where we were not allowed to go without tickets, but now people can watch movies online since the internet became cheaper.