Map Interface
The java.util.Map
interface represents a mapping between a key and a value. A key is an
object that you use to retrieve a value at a later date. Given a key and a value, you can store the value in a
Map
object. The
Collection
interface. Therefore it behaves a bit different from the rest of
the collection types.
Here is the topic that will cover in this post.
- Map Interface Declaration
- Map Implementations
- Map Interface Methods
- Exceptions
Map Interface Declaration
This interface is a member of java the java Collection framework. It is available since Java 1.2. Map is a generic interface and declared as show here:
Here, K specifies the type of keys, and V specifies the type of values.
Map Implementations
Map is an interface you need to instantiate a concrete implementation of the interface in order to use it. Several classes provide implementations of the map interfaces.
- java.util.AbstractMap
- java.util.EnumMap
- java.util.HashMap
- java.util.TreeMap
- java.util.WeakHashMap
- java.util.LinkedHashMap
- java.util.IdentityHashMap
- java.util.HashTable
- java.util.Properties
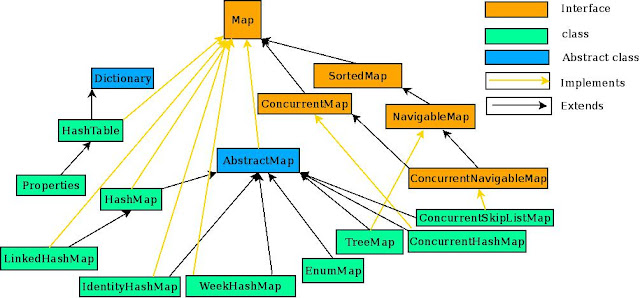
Each of these Map implementations behaves a little differently with respect to the order of the elements when
iterating the Map
.
HashMap
maps a key and a value. It does not guarantee any order of the elements stored internally in
the map.
TreeMap
also maps a key and a value. Furthermore it guarantees the order in which keys or values are
iterated - which is the sort order of the keys or values.
Example to create an instance of Map
Map hashMap = new HashMap(); Map treeMap = new TreeMap();
Map Interface Methods
Methods of map interface before java 8
Method | Description |
---|---|
int size() | Returns the number of key-value mappings in this map. |
boolean isEmpty() | Returns true if this map contains no key-value mappings. Otherwise, returns false. |
boolean containsKey(Object key) | Returns true if this map contains a mapping for the specified key. Otherwise, returns false. |
boolean containsValue(Object value) | Returns true if this map maps one or more keys to the specified value. Otherwise, returns false. |
V get(Object key) | Returns the value to which the specified key is mapped, or null if this map contains no
mapping for the key.
|
V put(K key, V value) | Puts an entry in the invoking map, overwriting any previous value associated with the key. The key and
value are key and value, respectively.
Returns null if the key did not already exist. Otherwise, the previous value linked to the
key is returned.
|
V remove(Object key) | Removes the mapping for a key from this map if it is present. Returns the value to which this map
previously associated the key, or null if the map contained no mapping for the key.
|
void putAll(Map<? extends K, ? extends V> m) | Copies all of the mappings from the specified map to this map. |
void clear() | Removes all of the mappings from this map. The map will be empty after this call returns. |
Set<K> keySet() | Returns a Set
view of the keys contained in this map. The set is backed by the map, so changes to the map are
reflected in the set, and vice-versa.
|
Collection<V> values() | Returns a Collection view of the values contained in this map. The
collection is backed by the map, so changes to the map are reflected in the collection, and vice-versa.
|
Set<Map.Entry<K, V>> entrySet() | Returns a Set
view of the mappings contained in this map. The set is backed by the map, so changes to the map are
reflected in the set, and vice-versa.
|
boolean equals(Object o) | Compares the specified object with this map for equality. Returns true if the given object is also a map and the two maps represent the same mappings. |
int hashCode() | Returns the hash code value for this map. |
Methods of map interface introduced in java 8
Method | Description |
---|---|
default V getOrDefault(Object key, V defaultValue) | Returns the value to which the specified key is mapped, or defaultValue if this map
contains no mapping for the key.
|
default void forEach(BiConsumer<? super K, ? super V> action) | Performs the given action for each entry in this map until all entries have been processed or the action throws an exception. A ConcurrentModificationException will be thrown if an element is removed during the process. |
default void replaceAll(BiFunction<? super K, ? super V, ? extends V> function) | Replaces each entry's value with the result of invoking the given function on that entry
until all entries have been processed or the
function throws an exception. Exceptions thrown by the function are relayed to the caller.
|
default V putIfAbsent(K key, V value) | If the specified key is not already associated with a value (or is mapped
to null ) associates it with the given value and returns
null , else returns the current value.
|
default boolean remove(Object key, Object value) | Removes the entry for the specified key only if it is currently mapped to the specified value. |
default boolean replace(K key, V oldValue, V newValue) | If the key/value pair specified by key and oldValue is in the invoking map, the value is replaced by newValue and true is returned. Otherwise false is returned. |
default V replace(K key, V value) | Replaces the entry for the specified key only if it is currently mapped to some value. |
default V computeIfAbsent(K key, Function<? super K, ? extends V> mappingFunction) | If the specified key is not already associated with a value (or is mapped to null ),
attempts to compute its value using the given mapping function and enters it into this map unless
null .
|
default V computeIfPresent(K key, BiFunction<? super K, ? super V, ? extends V> remappingFunction) | If key is in the map, a new value is constructed through
a call to remappingFunction and the new value replaces the old value
in the map. In this case, the new value is returned. If
the value returned by remappingFunction is null , the existing key and
value are removed from the map and null is returned.
|
default V compute(K key, BiFunction<? super K, ? super V, ? extends V> remappingFunction) | Calls remappingFunction to construct a new value. If remappingFunction returns
non-null, the new key/value pair is added to the
map or any preexisting pairing is removed, and the
new value is returned. If remappingFunction returns null , any
preexisting pairing is removed, and null is returned.
|
default V merge(K key, V value, BiFunction<? super V, ? super V, ? extends V> remappingFunction) | If the specified key is not already associated with a value or is
associated with null , associates it with the given non-null value.
Otherwise, replaces the associated value with the results of the given
remapping function, or removes if the result is null .
|
Exceptions
Map interface has many methods, and several methods throw below exceptions.
Exception | Description |
---|---|
ClassCastException | throws when an object is incompatible with the elements in a map. |
NullPointerException | thrown if an attempt is made to use a null object and null is not allowed in the map. |
UnsupportedOperationException | thrown when an attempt is made to change an unmodifiable map. |
IllegalArgumentException | thrown if an invalid argument is used. |
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
ReplyDeletefactorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.
factorial hundred In the last few days, the “factorial of 100” is one of the top subjects and a lot of maths geeks compute it using voice assistants such as Alexa, Shiri, etc.